Hi there,
Here’s how to setup a simple Timer Triggered Azure Function which, based on a scheduled cron setting, fetches a data-feed from a specified URL over https, and then stores it as a document into a pre-setup Azure CosmosDB that I won’t go into details with in this post.
First setup a Function App as described here: https://docs.microsoft.com/en-us/azure/azure-functions/functions-bindings-timer?tabs=javascript
Then add your function code logic, as for example (simplified but functional):
var https = require('https');
module.exports = function (context, myTimer) {
https.get("https://postman-echo.com/get?foo1=bar1&foo2=bar2", (res) => {
var responseData = "";
res.on("data", (chunk) => {
responseData += chunk;
});
res.on("end", () => {
context.log("Request succeeded");
context.log(responseData);
context.bindings.outputDoc = responseData;
context.res = {
"status": 200,
"body": responseData
};
context.done();
}
}).on("error", (error) => {
context.log("Request failed.");
context.log(error);
context.res = {
"status": 500,
"body": error
};
context.done();
});
setTimeout(function () {
context.log("Timed out, request took too long.");
context.done();
}, 1000);
};
Make sure to specify the desired cron settings on the function Trigger input, and select a valid and prepared CosmosDB as an output.
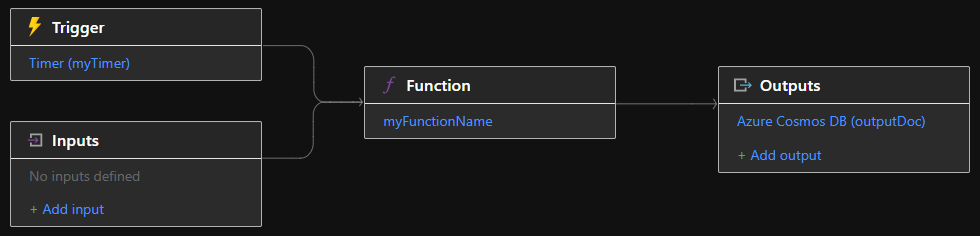
You should now see the data document inside your CosmosDB on a regular scheduled basis 🙂 – Enjoy!
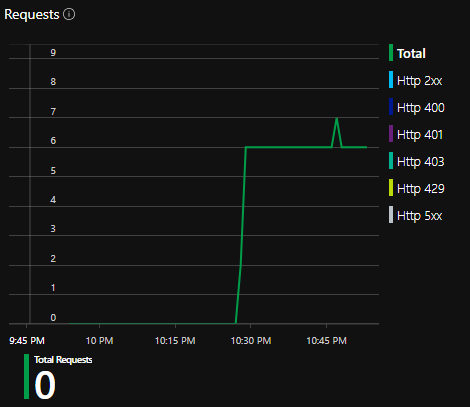